Annotate Profile
The below code snippet shows annotating the active profile element of an alignment. Here in the code profile is annotated with the station values and elevation values at interval which is hard coded in the code.
//Required References
using System;
using Bentley.DgnPlatformNET;
using Bentley.MstnPlatformNET;
using Bentley.CifNET.GeometryModel.SDK;
using Bentley.GeometryNET;
using Bentley.DgnPlatformNET.Elements;
using System.Diagnostics;
using Bentley.CifNET.LinearGeometry;
using Bentley.CifNET.Formatting;
public void AnnotateProfile()
{
try
{
//Get active dgn model
DgnModel model = Session.Instance.GetActiveDgnModel();
//Get active geometric model to get alignments
Bentley.CifNET.SDK.Edit.ConsensusConnectionEdit con = Bentley.CifNET.SDK.Edit.ConsensusConnectionEdit.GetActive();
GeometricModel geomModel = con.GetActiveGeometricModel();
//Get all alignments from current DGN
foreach (Alignment alignment in geomModel.Alignments)
{
if (!alignment.IsFinalElement)
continue;
//Get active profile of alignment
Profile profile = alignment.ActiveProfile;
LinearElement LinearEl = profile.ProfileGeometry;
double EndPosition = LinearEl.Length;
double Interval = 100;
double Length = 5;
//Create a line at interval along an alignment with the text annotation
for (double dStation = 0; EndPosition > dStation - Interval; dStation += Interval)
{
if (dStation > EndPosition)
dStation = EndPosition;
LinearPoint offsetPt0 = null, offsetPtLength = null;
offsetPt0 = LinearEl.GetPointAtDistanceOffset(dStation, 0);
offsetPtLength = LinearEl.GetPointAtDistanceOffset(dStation, -Length);
//User need unit conversion here on startPoint coordinates based on unit settings
DPoint3d startPoint = offsetPt0.Coordinates;
//User need unit conversion here on endPoint coordinates based on unit settings
DPoint3d endPoint = offsetPtLength.Coordinates;
//Create new line segment and add to model
DSegment3d segment = new DSegment3d(startPoint, endPoint);
LineElement lineElement = new Bentley.DgnPlatformNET.Elements.LineElement(model, null, segment);
lineElement.AddToModel();
DVector3d rotVector = segment.UnitTangent;
DMatrix3d rotMatrix = DMatrix3d.Rotation(2, rotVector.AngleXY);
//Create new DgnTextStyle element for annotation definition
DgnFile activeDgnFile = Bentley.MstnPlatformNET.Session.Instance.GetActiveDgnFile();
DgnTextStyle textStyle = DgnTextStyle.GetByName("Line Length Label", activeDgnFile);
if (null == textStyle)
{
textStyle = new DgnTextStyle("Line Length Label", activeDgnFile);
textStyle.SetProperty(TextStyleProperty.Width, 400D);
textStyle.SetProperty(TextStyleProperty.Height, 400D);
textStyle.Add(activeDgnFile);
}
//User need unit conversion here on dStation based on unit settings
double elevation = profile.ProfileGeometry.GetYAtX(dStation);
string elevationStr = FormatForDisplay.Distance(elevation, model, 3);
//User might need unit conversion for dStation based on unit settings
string textString = string.Format("sta = {0:F1}", dStation) + ", ele = " + elevationStr;
//New TextBlock for defining annotation
TextBlock textBlock = new TextBlock(textStyle, model);
textBlock.AppendText(textString);
textBlock.SetUserOrigin(startPoint);
textBlock.SetOrientation(rotMatrix);
//Add text annotation
TextElement textElement = (TextElement)TextElement.CreateElement(null, textBlock);
textElement.AddToModel();
}
}
}
catch (Exception ex)
{
Trace.WriteLine(ex.Message);
}
return;
}
Output
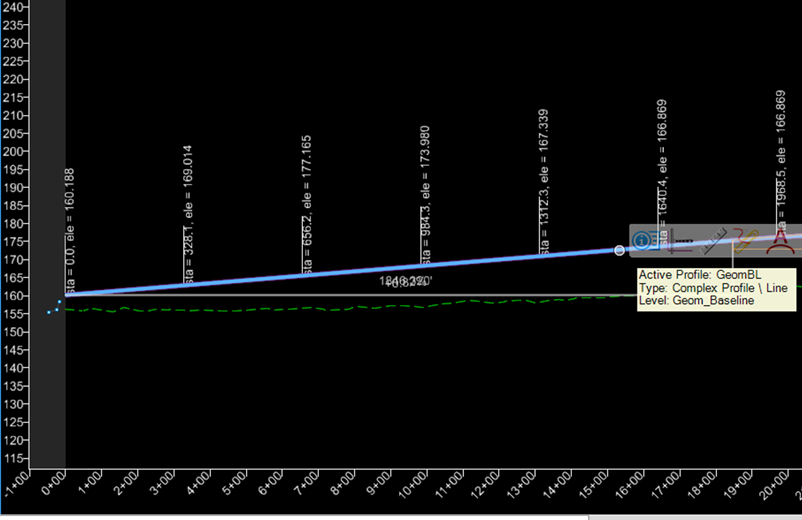